Lab 4 OO in Python
Aims
The aim of this lab is to investigate Object Orientation in python
- The principles of S.O.L.I.D
- Classes in Python
- OO Design principles
SOLID
Is A mnemonic to remember the first five principles of Object Oriented design
- Single responsibility
- Open-closed
- Liskov substitution
- Interface segregation
- Dependency inversion
The principles when applied together intend to make it more likely that a programmer will create a system that is easy to maintain and extend over time
Single Responsibility
- A class should do only one thing
- This should be entirely encapsulated in the class
- All services (methods) should be narrowly aligned to this responsibility
Open / Closed
- “software entities (classes, modules, functions, etc.) should be open for extension, but closed for modification”
- This means we shouldn’t modify the source but extend the code using inheritance
- This is sometimes know as “implementation inheritance”
- This can be done using Abstract base classes
Liskov substitution principle
- “objects in a program should be replaceable with instances of their subtypes without altering the correctness of that program”
- For a mutable objects “if S is a subtype of T, then objects of type T in a program may be replaced with objects of type S without altering any of the desirable properties of that program”
Interface segregation principle
- no client should be forced to depend on methods it does not use.
- ISP splits interfaces which are very large into smaller and more specific ones so that clients will only have to know about the methods that are of interest to them
- Sometimes called “role interfaces”
- This means we make classes “thin” not fat general purpose ones (which sometimes lead to software bloat)
Dependency inversion principle
- “One should Depend upon Abstractions. Do not depend upon concretions”
- High level modules should not depend on low level modules. Both should depend on abstractions.
- Abstractions should not depend upon details. Details should depend upon abstractions.
OO Design principles
DRY
Don’t repeat yourself (DRY, or sometimes do not repeat yourself) is a principle of software development aimed at reducing repetition of software patterns, replacing it with abstractions or using data normalization to avoid redundancy.
KISS
KISS is an acronym for “Keep it simple, stupid” as a design principle noted by the U.S. Navy in 1960. The KISS principle states that most systems work best if they are kept simple rather than made complicated; therefore, simplicity should be a key goal in design, and unnecessary complexity should be avoided.
YAGNI
You aren’t gonna need it (YAGNI) is a principle of extreme programming (XP) that states a programmer should not add functionality until deemed necessary. XP co-founder Ron Jeffries has written: “Always implement things when you actually need them, never when you just foresee that you need them.”
Textural analysis
The process of textural analysis is to read through a piece of text and identify the key words and phrases that are used.
Part of Speech | Component | Example |
---|---|---|
Proper Noun | Object / Instance | Jon |
Common Noun | Class | Person |
Adjective | Attribute (or member) | age |
Verb (doing) | Method | walk |
Verb (being) | State | is |
Verb (having) | composition | has |
This is a basic list and we can add many more, at it’s simplest level we can use this to identify the classes and methods in a piece of text.
Example
You are required to develop an image container that can be used to load and save images in a variety of formats. The image is specified by a width and height and the user should be able to set pixels within the image.
Internally the data needs to be stored in a simple RGBA type format stored as a single integer value. The user should be able to set the pixel data using a variety of methods including a single pixel, a row of pixels or the entire image.
Given the above description what classes and methods can we identify?
You are required to develop an image container that can be used to load and save images in a variety of formats. The image is specified by a width and height and the user should be able to set pixels within the image.
Internally the data needs to be stored in a simple RGBA type format stored as a single integer value. The user should be able to set the pixel data using a variety of methods including a single pixel, a row of pixels or the entire image.
We can now start to identify the classes and methods, we can also start to identify the attributes that the classes will need.
Class | Attributes | Methods |
---|---|---|
Image | width, height, format | load, save, set_pixel, set_row, set_all |
RGBA | r, g, b, a | set, get |
Format | name, extension |
We can now start to think about the relationships between the classes, we can see that the Image class has a composition relationship with the RGBA class as it contains a RGBA object. We can also see that the Image class has a composition relationship with the Format class as it contains a Format object.
But we can also simplify this more be ignoring the Format and setting this as an attribute of the Image class when saving (as most file I/O libraries hangle this).
Class Diagrams with UML
UML is a standardised way of describing classes and their relationships. It is a very large and complex standard but we will only be using a small subset of the features.
Class Diagrams
A class diagram is a static diagram that describes the structure of a system by showing the system’s classes, their attributes, operations (or methods), and the relationships among objects.
Unified Modelling Language (UML)
- Unified Modelling Language (UML) is a standardised general-purpose modelling language
- UML has a number of graphical element which allows us to describe various components of a software system in a standardised way
- We shall use the UML notation for class diagram when designing our classes as well as other elements from the UML 2.x standard
–
UML Classes
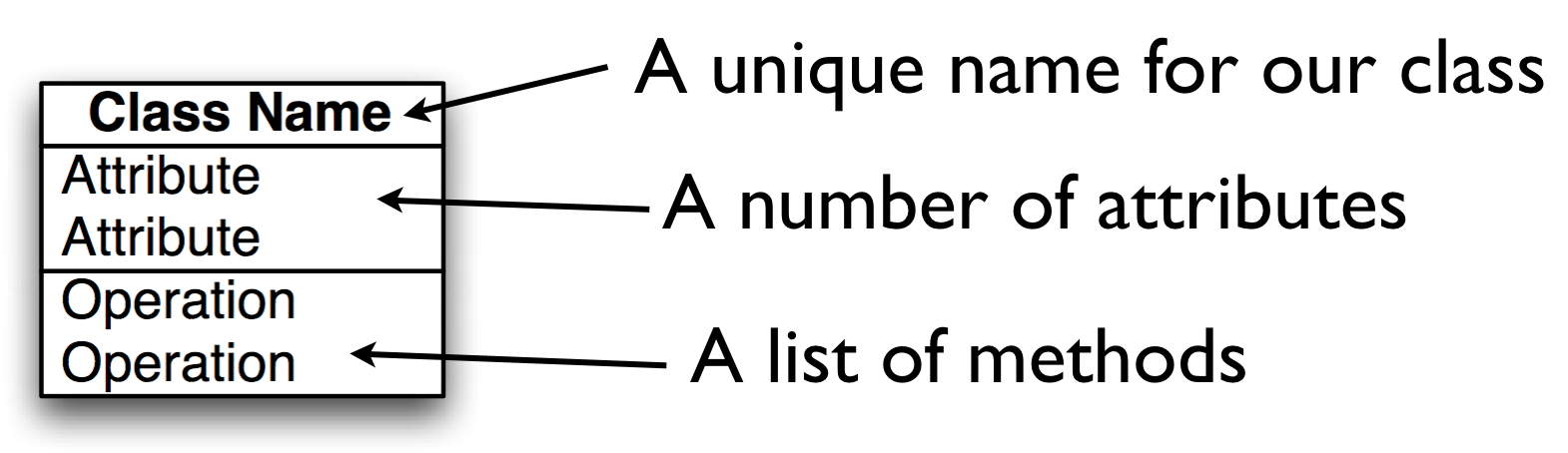
–
Specifying Attributes
- attributes are specified in the following way
[visibility] name : [data type]
- data type is any class type or built in data type
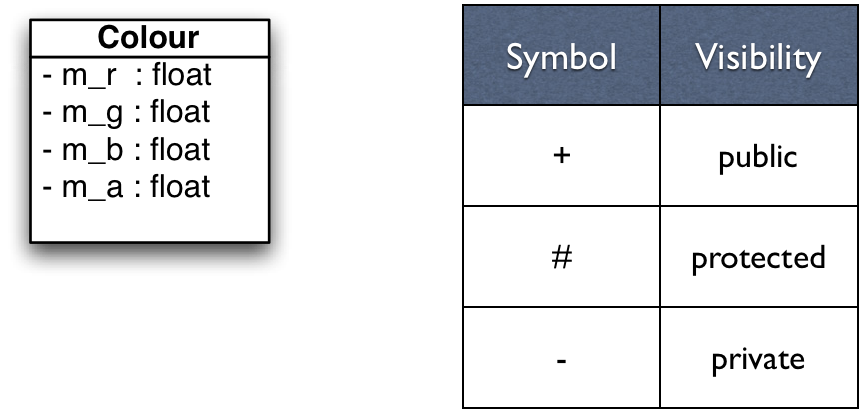
–
Specifying Methods
- Methods are defined as follows
[visibility] Name( [param] ) : [return type]
param := [name] : [data type]
- can have param,param …
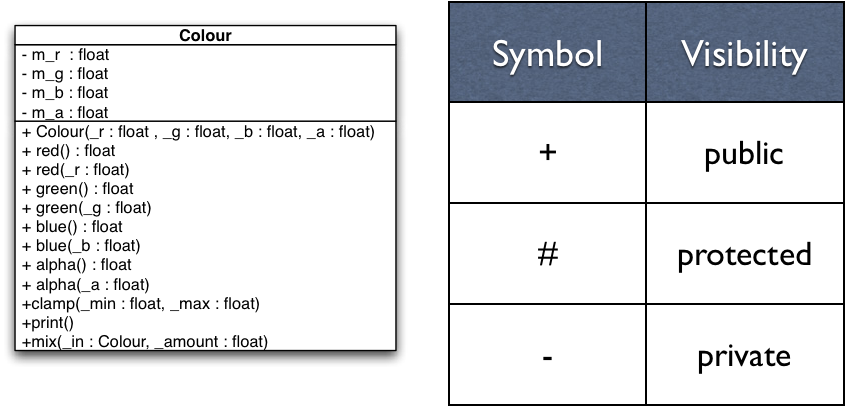